(Spring Boot) Gradle의 Profile(dev/production)을 구분지어보자.
(Spring Boot) get/post 리퀘스트를 다뤄보자.
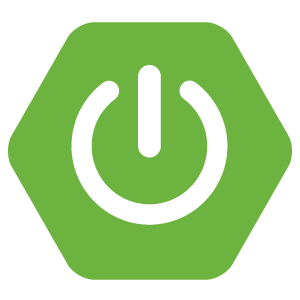
근본없이 궁금한 부분만 찾아서 공부하다보니 아직 정리가 덜 된 글이다 보니 그 점은 감안하고 보길 바란다.
컨트롤러를 만들자
Node.js(+Express)의 Router와 매우 유사한 것 같다.
URI와 http method, parameter만 매핑해주는 녀석이다.
1 | import org.springframework.web.bind.annotation.*; |
(Spring Boot) properties 값을 불러와보자
(Spring) 자바 빈즈 객체를 XML 파일로 관리하면서 DI하기 - property 태그
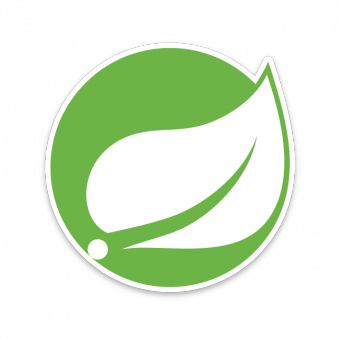
beans.xml 파일을 수정해보자.
1 | <?xml version="1.0" encoding="UTF-8"?> |
beans의 property는 getter/setter와 매핑이 된다.
Car.java를 수정해보자.
1 | // Car.java |
(Spring) 자바 빈즈 객체를 XML 파일로 관리하면서 DI하기 - @Autowired
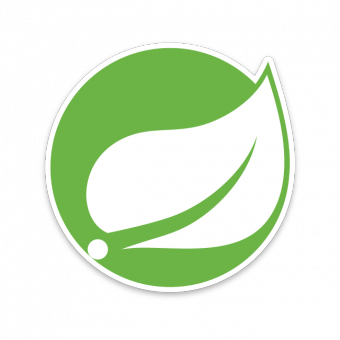
Car 클래스를 수정해보자.
1 | public class Car { |
1 | <?xml version="1.0" encoding="UTF-8"?> |
@Autowired 한 멤버 변수와 bean의 id가 매칭되는 걸 볼 수 있다.
또한 <context:annotation-config />을 추가해줘야하고, property 태그의 생략이 가능하다.
(Spring) 자바 빈즈 객체를 XML 파일로 관리하면서 DI하기 - 기본
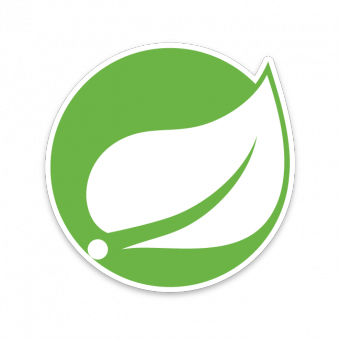
일반 자바를 가지고 DI를 해본 v3에서는 Car 클래스를 자바 빈즈 스펙을 제대로 준수해서 만들지 않았다.
따라서 한 번 자바 빈즈 스펙에 맞춰 바꿔보자.
1 | public class Car { |
getter/setter와 기본 생성자가 있어야 자바 빈즈 스펙을 준수한 것이었는데 저번에는 기본 생성자가 없어서 추가했다.
그럼 이제 beans.xml 파일을 만들고 자바 빈즈 객체들을 등록해보자.
1 | <?xml version="1.0" encoding="UTF-8"?> |
Dependency Injection(의존성 주입)을 알아보자 - 생성자 함수
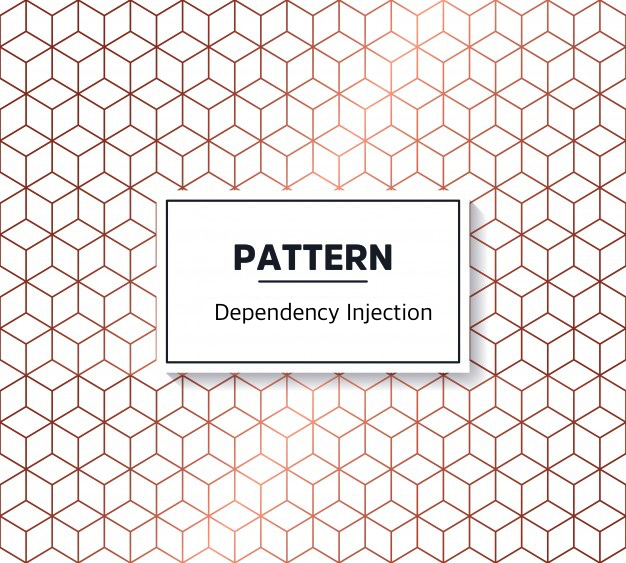
v1에는 재사용 가능한 코드가 있음에도 불구하고 미묘(?)한 차이 때문에 계속 각국의 타이어를 장착한 자동차 클래스를 만들어야하는 단점이 있었다.
이는 자동차를 만들 때 이미 타이어를 만드는 방법이 결정되어 있기 때문에 발생하는 문제이다.
(**자동차(전체)**가 **타이어(부분)**에 의존하고 있는 코드)
즉, 자동차를 만들 때 타이어를 만드는 방법을 결정하면 되는 사항이다.
(**의존하는 부분(타이어)**을 **전체(자동차)**에 주입시키는 패턴)
1 | // Tire.java |
1 | // KoreanTire.java |
1 | // AmericanTrie.java |
Dependency Injection(의존성 주입)을 알아보자 - setter
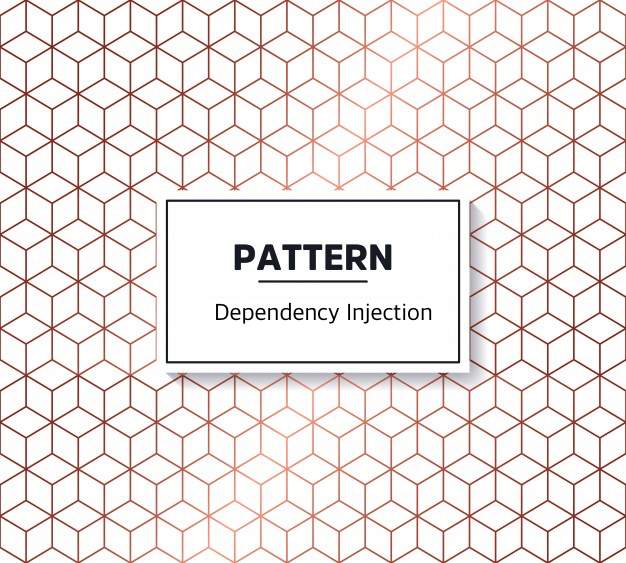
v2에는 자동차를 생산할 때 어떤 타이어를 만들지 정할 수 있고 새로운 타이어로 교체도 가능했다.
하지만 올바른 값이 들어왔는지 유효성 검사할 방법이 없다.
사실 변경할 수는 있지만 안전하지 않고 그닥 권장하는 방법이 아니다.
1 | // Car.java |
1 | // Driver.java |
setter를 사용해 좀 더 안전하게(?) 타이어를 교체할 수 있게 되었다.
대부분 getter/setter를 사용하는 이유는 아마 다음과 같을 것이다.
Dependency Injection(의존성 주입)을 알아보자 - 막코딩 하기
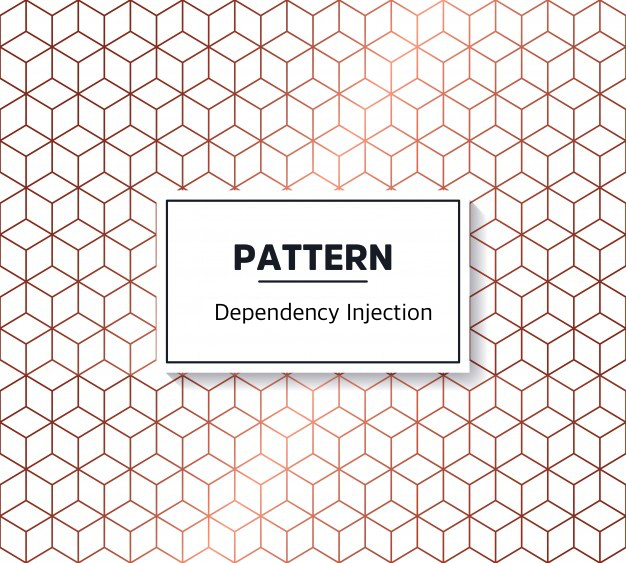
이 글은 의존성 주입을 전혀 적용하지 않은, 의존성 주입이 뭔지 모르는 상태로 짠 코드이다.
우선 문제점을 먼저 파악해봐야 뭐가 되지 않을까 싶어서 막코딩을 해봤다고 가정해보자.
우선 미국산 타이어가 장착된 자동차, 한국산 타이어가 장착된 자동차를 만들어야한다고 생각해보자.
그럼 우선 미국산, 한국산 타이어 클래스 두 개가 필요할 것이다.
1 | // KoreanTire.java |
1 | // AmericanTire.java |
그리고 각각 미국산 타이어를 장착한 자동차, 한국산 타이어를 장착한 자동차 클래스 두 개를 만들면 끝난다.